Here is how you can create a .dll using cpp and then use it inside of Python. It is not as complicated as one would think.
Create a new Win32 Console Application under Visual C++
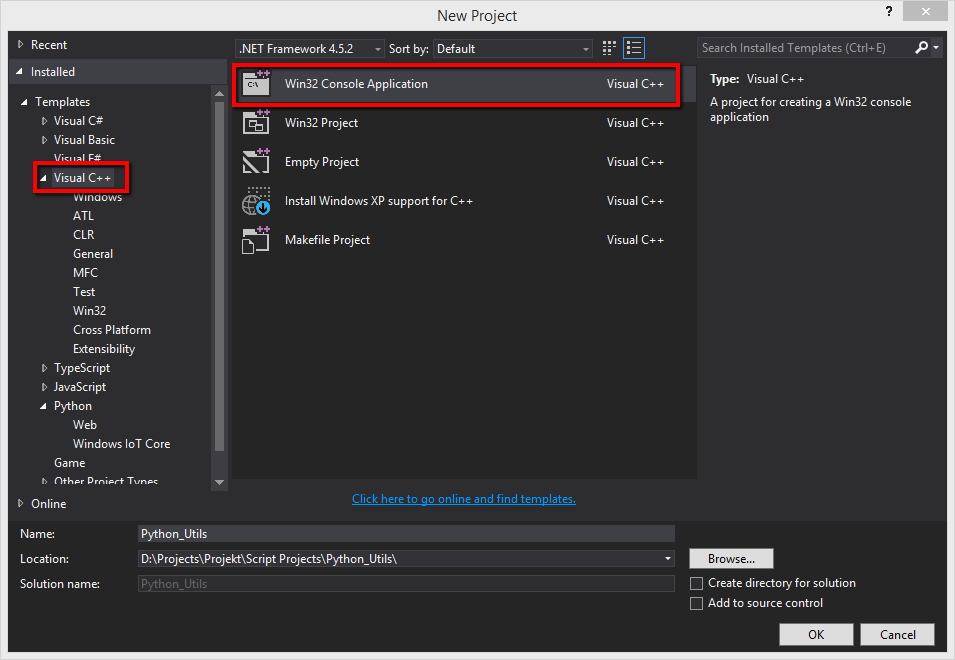
I have named the file to Python_Utils, since that is what I want to use it for.
Right click Header Files folder and Add New Item
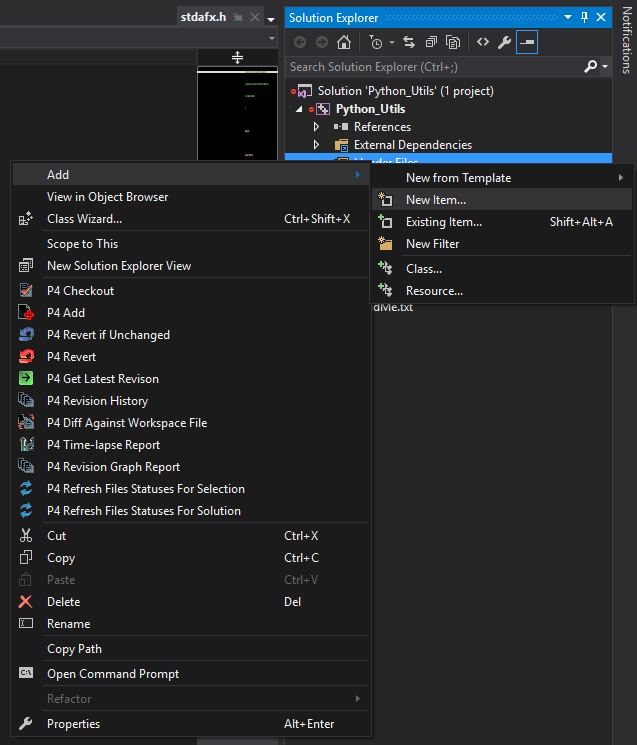
Then name that file. I named it interface.
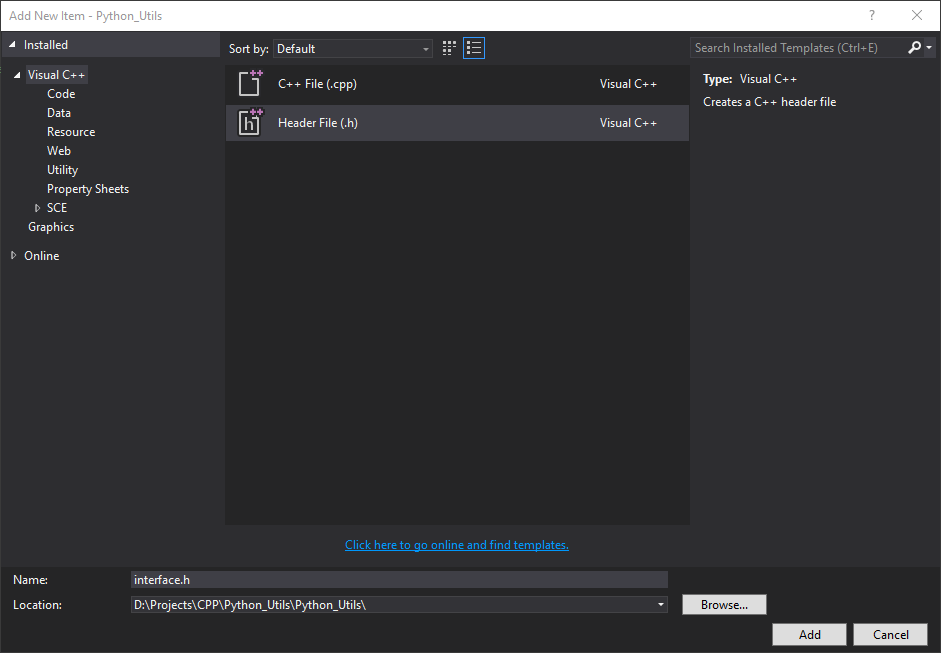
Now you should have a few header files and cpp files. Remove the ones you are not interested in.
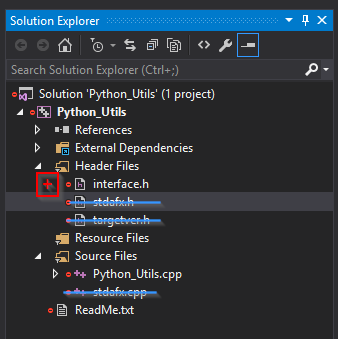
Inside the “your name of the file”.cpp (in this case Python_Utils.cpp) don’t forget to #include the newly created header file.
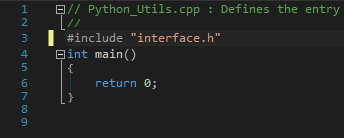
Now you need to go into settings for this project.
Go to Build –> Configuration Manager
Change it to look like this.
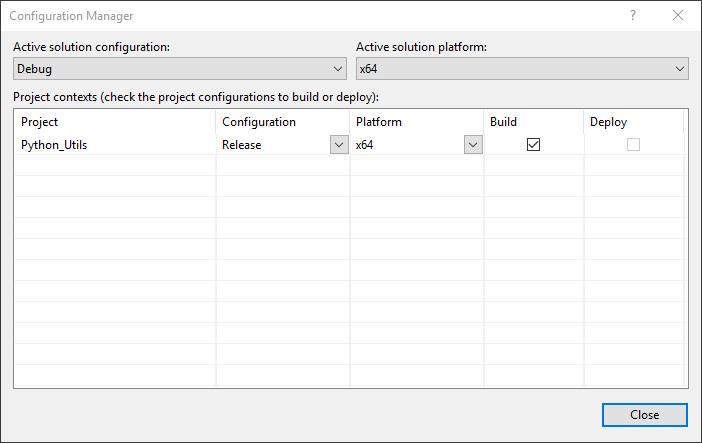
There are some more settings that needs to be changed.
Right click (your name of the file)
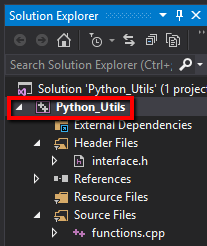
Select general and change Configuration type to Dynamic Library (.dll)
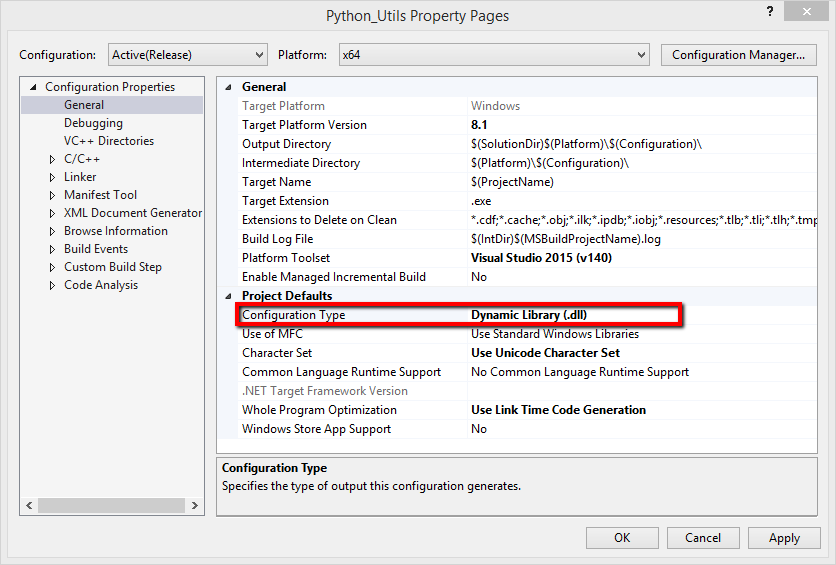
Open up C/C++ section and select “Precompiled Headers” and changed to Not Using Precompiled Headers.
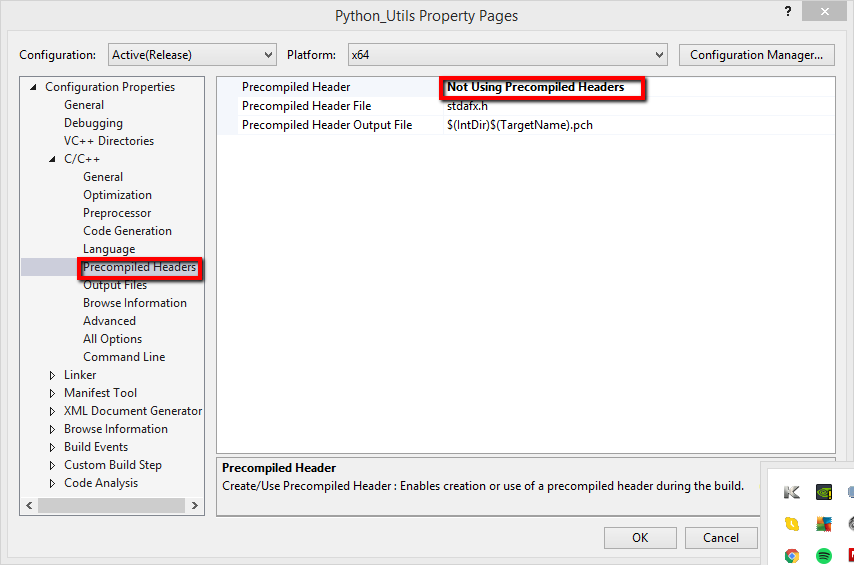
Hit apply to have your changes take affect.
Try to build your dll.
If you see this error

You will have to change this one. General –> Target Platform Version. I had to change it to 10.0.10586.0
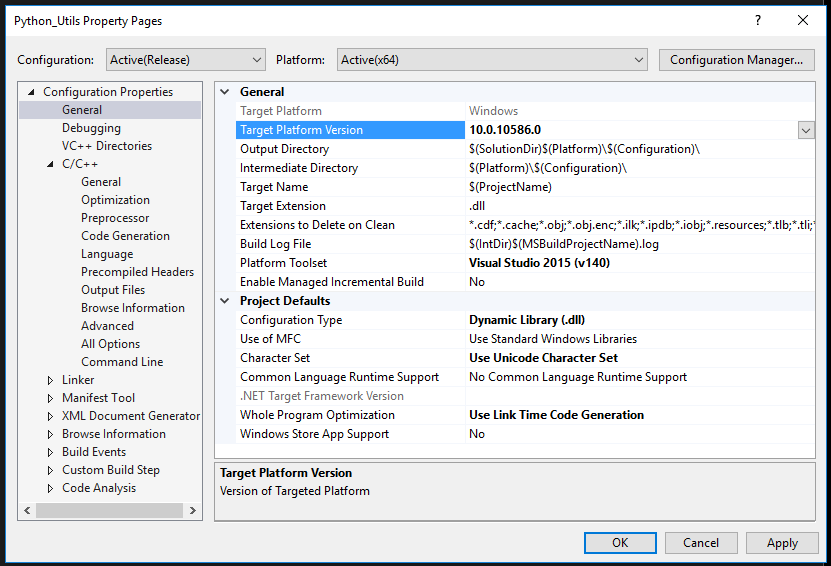
Now hopefully you should be able to build your dll. But of course you will not be able to access anything in this file at the moment. You will have to expose this in the .h file.
So lets create a simple method that returns the value of 2 int values.
Add this to your .cpp file.
int sum(int a, int b) { return a + b; }
In your .h file you should add this.
extern "C" __declspec(dllexport) int __cdecl sum( int, int );
Rebuild your .dll.
You can now test your .dll by copying this .dll into one of your python projects and include it like this.
from ctypes import cdll utils = cdll.LoadLibrary("Python_Utils")
Now you can try it out by just printing this
print( utils.sum(5,6) )
To it a bit easier when building your .dll you can make it copy itself after it has been built into your python project by adding this to the post build step.
Go to properties –> Build Events –> Post-Build Event
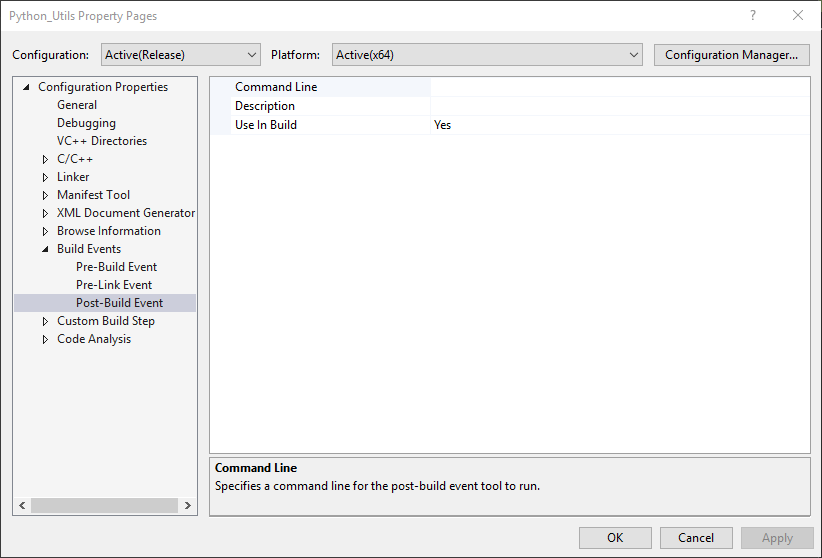
Click Edit in the Command Line and add this.
copy /Y “$(TargetDir)$(ProjectName).dll” “Full_Path_To_Your_Python_Project\Your_DLL_Name.dll”
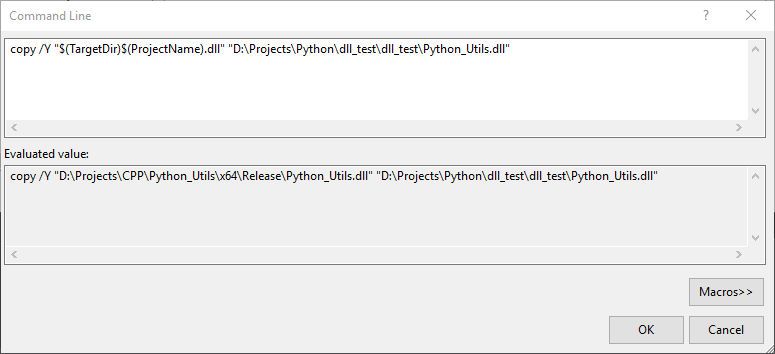
Should look like this now.
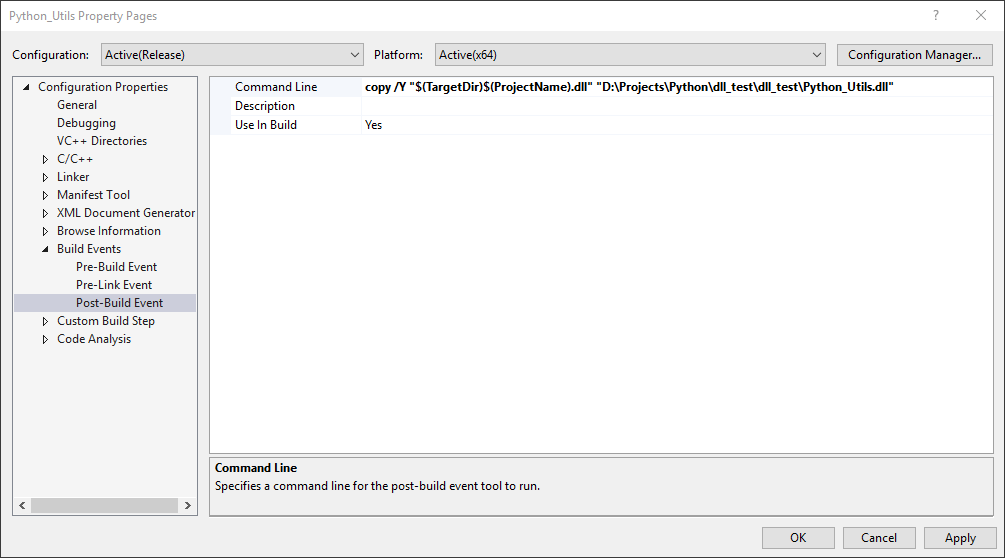
Now every time you build your .dll it will copy itself into your python project.
Leave a Reply